Earth Engine for gathering data¶
Google Earth Engine is a powerful tool for geospatial data analysis. Here, we will discuss how to use its Python API for gathering data from Landsat, Sentinel, and NAIP imagery.
To start off with, request access to Google Earth Engine at: . This may take some time. Once approved, install the Python API and get credentials for using the API following these instructions. Be sure to try out the test code they suggest to make sure Earth Engine is running successfully):
import ee
# Initialize the Earth Engine module.
ee.Initialize()
# Print metadata for a DEM dataset.
print(ee.Image('USGS/SRTMGL1_003').getInfo())
{'type': 'Image', 'bands': [{'id': 'elevation', 'data_type': {'type': 'PixelType', 'precision': 'int', 'min': -32768, 'max': 32767}, 'dimensions': [1296001, 417601], 'crs': 'EPSG:4326', 'crs_transform': [0.0002777777777777778, 0, -180.0001388888889, 0, -0.0002777777777777778, 60.00013888888889]}], 'id': 'USGS/SRTMGL1_003', 'version': 1641990767055141, 'properties': {'system:visualization_0_min': '0.0', 'type_name': 'Image', 'keywords': ['dem', 'elevation', 'geophysical', 'nasa', 'srtm', 'topography', 'usgs'], 'thumb': 'https://mw1.google.com/ges/dd/images/SRTM90_V4_thumb.png', 'description': '<p>The Shuttle Radar Topography Mission (SRTM, see <a href="https://onlinelibrary.wiley.com/doi/10.1029/2005RG000183/full">Farr\net al. 2007</a>)\ndigital elevation data is an international research effort that\nobtained digital elevation models on a near-global scale. This\nSRTM V3 product (SRTM Plus) is provided by NASA JPL\nat a resolution of 1 arc-second (approximately 30m).</p><p>This dataset has undergone a void-filling process using open-source data\n(ASTER GDEM2, GMTED2010, and NED), as opposed to other versions that\ncontain voids or have been void-filled with commercial sources.\nFor more information on the different versions see the\n<a href="https://lpdaac.usgs.gov/documents/13/SRTM_Quick_Guide.pdf">SRTM Quick Guide</a>.</p><p>Documentation:</p><ul><li><p><a href="https://lpdaac.usgs.gov/documents/179/SRTM_User_Guide_V3.pdf">User's Guide</a></p></li><li><p><a href="https://lpdaac.usgs.gov/documents/13/SRTM_Quick_Guide.pdf">General Documentation</a></p></li><li><p><a href="https://doi.org/10.1029/2005RG000183">Algorithm Theoretical Basis Document (ATBD)</a></p></li></ul><p><b>Provider: <a href="https://cmr.earthdata.nasa.gov/search/concepts/C1000000240-LPDAAC_ECS.html">NASA / USGS / JPL-Caltech</a></b><br><p><b>Bands</b><table class="eecat"><tr><th scope="col">Name</th><th scope="col">Description</th></tr><tr><td>elevation</td><td><p>Elevation</p></td></tr></table><p><b>Terms of Use</b><br><p>Unless otherwise noted, images and video on JPL public\nweb sites (public sites ending with a jpl.nasa.gov address) may\nbe used for any purpose without prior permission. For more information\nand exceptions visit the <a href="https://www.jpl.nasa.gov/imagepolicy/">JPL Image Use Policy site</a>.</p><p><b>Suggested citation(s)</b><ul><li><p>Farr, T.G., Rosen, P.A., Caro, E., Crippen, R., Duren, R., Hensley,\nS., Kobrick, M., Paller, M., Rodriguez, E., Roth, L., Seal, D.,\nShaffer, S., Shimada, J., Umland, J., Werner, M., Oskin, M., Burbank,\nD., and Alsdorf, D.E., 2007, The shuttle radar topography mission:\nReviews of Geophysics, v. 45, no. 2, RG2004, at\n<a href="https://doi.org/10.1029/2005RG000183">https://doi.org/10.1029/2005RG000183</a>.</p></li></ul><style>\n table.eecat {\n border: 1px solid black;\n border-collapse: collapse;\n font-size: 13px;\n }\n table.eecat td, tr, th {\n text-align: left; vertical-align: top;\n border: 1px solid gray; padding: 3px;\n }\n td.nobreak { white-space: nowrap; }\n</style>', 'source_tags': ['nasa', 'usgs'], 'visualization_0_max': '6000.0', 'title': 'NASA SRTM Digital Elevation 30m', 'product_tags': ['srtm', 'elevation', 'topography', 'dem', 'geophysical'], 'provider': 'NASA / USGS / JPL-Caltech', 'visualization_0_min': '0.0', 'visualization_0_name': 'Elevation', 'date_range': [950227200000, 951177600000], 'system:visualization_0_gamma': '1.6', 'period': 0, 'system:visualization_0_bands': 'elevation', 'provider_url': 'https://cmr.earthdata.nasa.gov/search/concepts/C1000000240-LPDAAC_ECS.html', 'visualization_0_gamma': '1.6', 'sample': 'https://mw1.google.com/ges/dd/images/SRTM90_V4_sample.png', 'tags': ['dem', 'elevation', 'geophysical', 'nasa', 'srtm', 'topography', 'usgs'], 'system:visualization_0_max': '6000.0', 'system:visualization_0_name': 'Elevation', 'system:asset_size': 132792638252, 'visualization_0_bands': 'elevation'}}
import json
print(json.dumps(ee.Image('USGS/SRTMGL1_003').getInfo(), indent=3, sort_keys=True))
{
"bands": [
{
"crs": "EPSG:4326",
"crs_transform": [
0.0002777777777777778,
0,
-180.0001388888889,
0,
-0.0002777777777777778,
60.00013888888889
],
"data_type": {
"max": 32767,
"min": -32768,
"precision": "int",
"type": "PixelType"
},
"dimensions": [
1296001,
417601
],
"id": "elevation"
}
],
"id": "USGS/SRTMGL1_003",
"properties": {
"date_range": [
950227200000,
951177600000
],
"description": "<p>The Shuttle Radar Topography Mission (SRTM, see <a href=\"https://onlinelibrary.wiley.com/doi/10.1029/2005RG000183/full\">Farr\net al. 2007</a>)\ndigital elevation data is an international research effort that\nobtained digital elevation models on a near-global scale. This\nSRTM V3 product (SRTM Plus) is provided by NASA JPL\nat a resolution of 1 arc-second (approximately 30m).</p><p>This dataset has undergone a void-filling process using open-source data\n(ASTER GDEM2, GMTED2010, and NED), as opposed to other versions that\ncontain voids or have been void-filled with commercial sources.\nFor more information on the different versions see the\n<a href=\"https://lpdaac.usgs.gov/documents/13/SRTM_Quick_Guide.pdf\">SRTM Quick Guide</a>.</p><p>Documentation:</p><ul><li><p><a href=\"https://lpdaac.usgs.gov/documents/179/SRTM_User_Guide_V3.pdf\">User's Guide</a></p></li><li><p><a href=\"https://lpdaac.usgs.gov/documents/13/SRTM_Quick_Guide.pdf\">General Documentation</a></p></li><li><p><a href=\"https://doi.org/10.1029/2005RG000183\">Algorithm Theoretical Basis Document (ATBD)</a></p></li></ul><p><b>Provider: <a href=\"https://cmr.earthdata.nasa.gov/search/concepts/C1000000240-LPDAAC_ECS.html\">NASA / USGS / JPL-Caltech</a></b><br><p><b>Bands</b><table class=\"eecat\"><tr><th scope=\"col\">Name</th><th scope=\"col\">Description</th></tr><tr><td>elevation</td><td><p>Elevation</p></td></tr></table><p><b>Terms of Use</b><br><p>Unless otherwise noted, images and video on JPL public\nweb sites (public sites ending with a jpl.nasa.gov address) may\nbe used for any purpose without prior permission. For more information\nand exceptions visit the <a href=\"https://www.jpl.nasa.gov/imagepolicy/\">JPL Image Use Policy site</a>.</p><p><b>Suggested citation(s)</b><ul><li><p>Farr, T.G., Rosen, P.A., Caro, E., Crippen, R., Duren, R., Hensley,\nS., Kobrick, M., Paller, M., Rodriguez, E., Roth, L., Seal, D.,\nShaffer, S., Shimada, J., Umland, J., Werner, M., Oskin, M., Burbank,\nD., and Alsdorf, D.E., 2007, The shuttle radar topography mission:\nReviews of Geophysics, v. 45, no. 2, RG2004, at\n<a href=\"https://doi.org/10.1029/2005RG000183\">https://doi.org/10.1029/2005RG000183</a>.</p></li></ul><style>\n table.eecat {\n border: 1px solid black;\n border-collapse: collapse;\n font-size: 13px;\n }\n table.eecat td, tr, th {\n text-align: left; vertical-align: top;\n border: 1px solid gray; padding: 3px;\n }\n td.nobreak { white-space: nowrap; }\n</style>",
"keywords": [
"dem",
"elevation",
"geophysical",
"nasa",
"srtm",
"topography",
"usgs"
],
"period": 0,
"product_tags": [
"srtm",
"elevation",
"topography",
"dem",
"geophysical"
],
"provider": "NASA / USGS / JPL-Caltech",
"provider_url": "https://cmr.earthdata.nasa.gov/search/concepts/C1000000240-LPDAAC_ECS.html",
"sample": "https://mw1.google.com/ges/dd/images/SRTM90_V4_sample.png",
"source_tags": [
"nasa",
"usgs"
],
"system:asset_size": 132792638252,
"system:visualization_0_bands": "elevation",
"system:visualization_0_gamma": "1.6",
"system:visualization_0_max": "6000.0",
"system:visualization_0_min": "0.0",
"system:visualization_0_name": "Elevation",
"tags": [
"dem",
"elevation",
"geophysical",
"nasa",
"srtm",
"topography",
"usgs"
],
"thumb": "https://mw1.google.com/ges/dd/images/SRTM90_V4_thumb.png",
"title": "NASA SRTM Digital Elevation 30m",
"type_name": "Image",
"visualization_0_bands": "elevation",
"visualization_0_gamma": "1.6",
"visualization_0_max": "6000.0",
"visualization_0_min": "0.0",
"visualization_0_name": "Elevation"
},
"type": "Image",
"version": 1641990767055141
}
If the above code yields output, you’ll know you’re ready for to go!
Selecting imagery¶
from geetools import batch
def download_NAIP_toLocal(bbox, name, scale=1):
"""
downloads NAIP imagery from the specified bounding box and saves it as `name`
"""
AOI = ee.Geometry.Rectangle(list(bbox), "EPSG:4326", False)
collection = (
ee.ImageCollection("USDA/NAIP/DOQQ")
.filterDate("2010-01-01", "2019-01-01")
.filterBounds(AOI)
.select(['R', 'G', 'B'])
)
image = ee.Image(collection.mosaic()).clip(AOI)
batch.image.toLocal(image, name, scale=scale, region=AOI)
lat = [35.998708946524324, 36.003257110633896]
lon = [-78.94764325291365, -78.9412917819664]
bbox = [lon[0], lat[0], lon[1], lat[1]]
name = 'naipsample'
download_NAIP_toLocal(bbox, name, scale=1)
# Unzip the files
import zipfile
path_to_zip_file = 'naipsample.zip'
directory_to_extract_to = 'naipsample'
with zipfile.ZipFile(path_to_zip_file, 'r') as zip_ref:
zip_ref.extractall(directory_to_extract_to)
# Combine the files:
import rasterio
def stack_bands(name, outname):
file_list = [name + '.R.tif', name + '.G.tif', name + '.B.tif']
# Read metadata of first file
with rasterio.open(file_list[0]) as src0:
meta = src0.meta
# Update meta to reflect the number of layers
meta.update(count = len(file_list))
# Read each layer and write it to stack
with rasterio.open(outname, 'w', **meta) as dst:
for id, layer in enumerate(file_list, start=1):
with rasterio.open(layer) as src1:
dst.write_band(id, src1.read(1))
outname = "stacked_sample.tif"
stack_bands('naipsample/download',outname)
# Plot the example
%config InlineBackend.figure_format = 'retina'
import matplotlib.pyplot as plt
from rasterio.plot import show
fig, ax = plt.subplots(num='main', figsize=(6,6))
from rasterio.plot import show
data = rasterio.open(outname)
show(data.read([1,2,3]).astype('float')/255, transform=data.transform, ax=ax)
<AxesSubplot:>
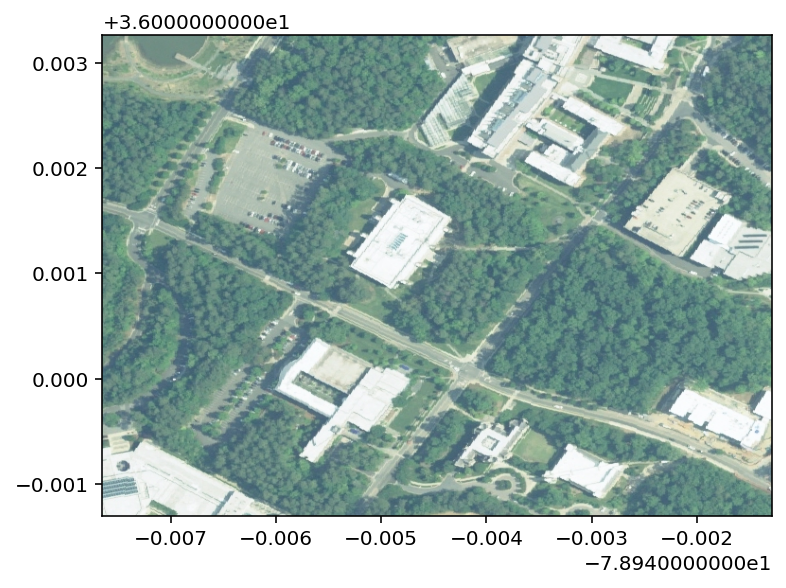
from geetools import batch
def download_S2_toLocal(bbox, name, scale=1):
"""
downloads Sentinel 2 imagery from the specified bounding box and saves it as `name`
"""
AOI = ee.Geometry.Rectangle(list(bbox), "EPSG:4326", False)
collection = (
ee.ImageCollection("COPERNICUS/S2_SR")
.filterDate('2020-01-01', '2020-01-30')
.filter(ee.Filter.lt('CLOUDY_PIXEL_PERCENTAGE',20))
.filterBounds(AOI)
.select(['B4', 'B3', 'B2'])
)
image = ee.Image(collection.mosaic()).clip(AOI)
batch.image.toLocal(image, name, scale=scale, region=AOI)
lat = [35.998708946524324, 36.003257110633896]
lon = [-78.94764325291365, -78.9412917819664]
bbox = [lon[0], lat[0], lon[1], lat[1]]
name = 's2sample'
download_S2_toLocal(bbox, name, scale=1)
import zipfile
path_to_zip_file = 's2sample.zip'
directory_to_extract_to = 's2sample'
with zipfile.ZipFile(path_to_zip_file, 'r') as zip_ref:
zip_ref.extractall(directory_to_extract_to)
import rasterio
def stack_bands_s2(name, outname):
file_list = [name + '.B4.tif', name + '.B3.tif', name + '.B2.tif']
# Read metadata of first file
with rasterio.open(file_list[0]) as src0:
meta = src0.meta
# Update meta to reflect the number of layers
meta.update(count = len(file_list))
# Read each layer and write it to stack
with rasterio.open(outname, 'w', **meta) as dst:
for id, layer in enumerate(file_list, start=1):
with rasterio.open(layer) as src1:
dst.write_band(id, src1.read(1))
outname = "stacked_s2_sample.tif"
stack_bands_s2('s2sample/download',outname)
# Plot the example
%config InlineBackend.figure_format = 'retina'
import matplotlib.pyplot as plt
from rasterio.plot import show
fig, ax = plt.subplots(num='main', figsize=(6,6))
from rasterio.plot import show
data = rasterio.open(outname)
show(data.read([1,2,3]).astype('float')/4096, transform=data.transform, ax=ax)
# show(data.read([1,2,3]).astype('float')/255, transform=data.transform, ax=ax)
Clipping input data to the valid range for imshow with RGB data ([0..1] for floats or [0..255] for integers).
<AxesSubplot:>
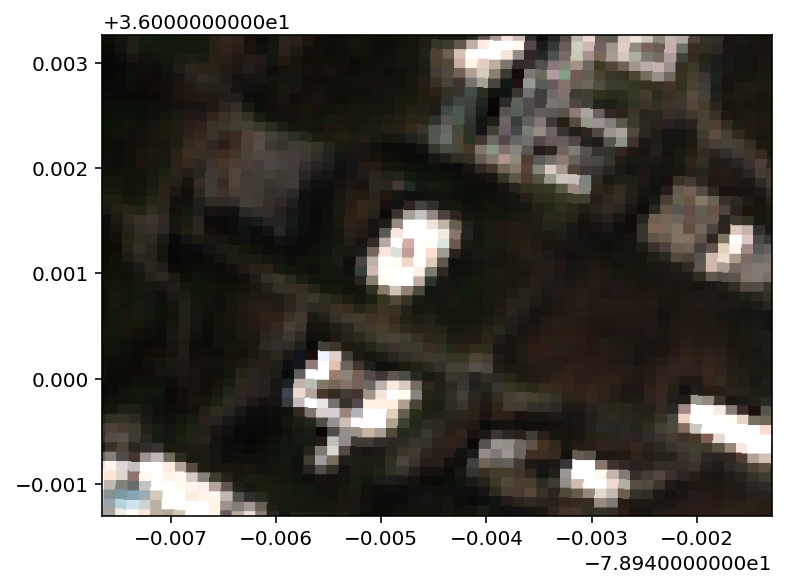
from geetools import batch
lat = [35.998708946524324, 36.003257110633896]
lon = [-78.94764325291365, -78.9412917819664]
bbox = [lon[0], lat[0], lon[1], lat[1]]
name = 's2sample'
AOI = ee.Geometry.Rectangle(list(bbox), "EPSG:4326", False)
collection = ee.ImageCollection("COPERNICUS/S2_SR").filterDate("2010-01-01", "2019-01-01").filterBounds(AOI).select(['B4', 'B3', 'B2'])
collection
<ee.imagecollection.ImageCollection at 0x16f497880>
image = ee.Image(collection.mosaic()).clip(AOI)
# batch.image.toLocal(image, name, scale=scale, region=AOI)